Integration of payment systems in online casinos – Step by step
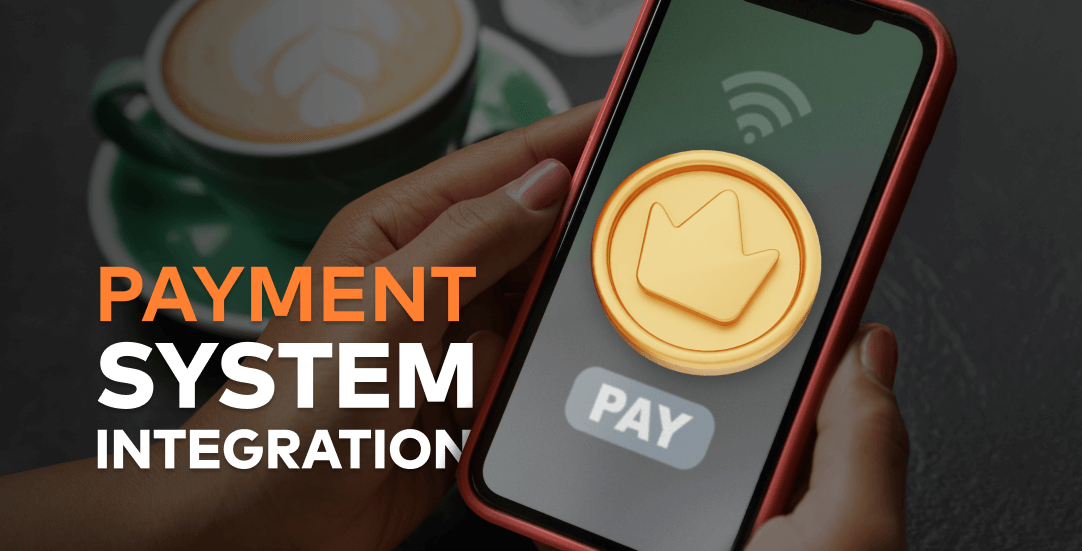
Online casinos thrive on providing fast, secure, and seamless experiences to their users. In today’s digital background, integrating efficient payment systems is not only a technical necessity but also a strategic advantage. This guide walks you through the integration process, offering insights into development practices, practical examples, and real-world considerations to ensure your platform remains competitive and compliant.
Requirement analysis and planning
Before starting any integration, conduct a comprehensive analysis of your platform’s financial requirements. This involves understanding transaction volumes, user demographics, regulatory requirements, and future scalability needs. Note that provider selection often depends on the region in which your casino operates. For example, regulations may differ significantly between regions—while one country may have general guidelines, specific areas (like Ontario in Canada) enforce stricter rules.
In this phase, map out the existing architecture, identify gaps, and plan for future growth. Research current market trends and regulatory requirements, such as AML (Anti-Money Laundering) and KYC (Know Your Customer), which are critical for ensuring compliance. Additionally, consider incorporating support for emerging payment options, including cryptocurrencies, to attract a broader user base.
Selecting the right payment provider
Choosing the right payment provider is pivotal. Providers vary by region, and their services must align with local regulations as well as international standards. Factors to evaluate include:
- regional compliance – ensure that the provider meets regulatory requirements specific to your market (e.g., stricter rules in Ontario, Canada),
- security measures – look for robust AML and KYC protocols,
- support for multiple payment methods – in addition to traditional payments, having support for cryptocurrencies can be a significant advantage,
- provider libraries – some providers offer their own SDKs or libraries (e.g., Nuvei’s PHP SDK) to simplify integration. If a dedicated library is available, it should be used to handle communication with their API. If not, you’ll need to program the API requests manually according to the provider’s documentation,
- OOP and design patterns – from a code perspective, it’s crucial to encapsulate each provider’s implementation behind a common interface. This can be achieved using design patterns like the strategy pattern, ensuring your system remains modular and maintainable.
API integration and payment gateway implementation
At the core of any payment system is the communication between your casino’s backend and external financial networks, typically achieved through APIs. A well-designed payment gateway ensures secure data transfer and reliable transaction processing.
Planning the API architecture
Develop a modular API architecture with clearly defined endpoints for various transactions. Use secure authentication methods (e.g., API keys or OAuth tokens) and establish comprehensive error handling and logging. This logging is critical—not only for debugging purposes but also to record detailed information on every successful or unsuccessful transaction.
Implementing the gateway
Below is a very basic example in Node.js. This code demonstrates how to send a payment request via an API. Note that in real-world applications, many providers offer dedicated libraries that handle these requests more elegantly.
const axios = require('axios'); async function processPayment(paymentData) { try { const response = await axios.post('https://api.paymentprovider.com/process', paymentData, { headers: { 'Content-Type': 'application/json', 'Authorization': 'Bearer YOUR_API_KEY' } }); if (response.data.status === 'success') { console.log('Transaction completed successfully'); } else { console.log('Payment processing error:', response.data.message); } } catch (error) { console.error('API call error:', error.message); } } // Example payment data (basic example) const paymentData = { amount: 100, currency: 'USD', method: 'credit_card', cardDetails: { number: '4111111111111111', expiry: '12/25', cvv: '123' } }; processPayment(paymentData);
Note: This example is for demonstration purposes only. In a production system, consider using the provider’s solutions if available, and encapsulate API calls within classes that adhere to a common interface.
Development and code optimization
Thorough testing is essential. Providers often offer test APIs or staging environments so developers can simulate transactions without affecting live operations. Remember:
- unit tests – these should focus solely on your code and not send real requests to external providers,
- integration testing – use test APIs provided by the payment provider to simulate real-world scenarios,
- load and stress testing – ensure that the system handles peak loads effectively.
Managing payment risks
Security is paramount. Implement a robust risk management framework that includes:
- advanced fraud detection – use algorithms and real-time analytics to detect suspicious activity,
- compliance measures – regularly update systems to adhere to AML and KYC regulations,
- detailed logging – capture every transaction detail to aid in audits and incident response,
- manual confirmation – for transactions that require human oversight, maintain a “pending” status until confirmed.
These measures not only protect user data but also build trust with your users, ensuring that all transactions are processed securely.
How are payment methods evolving in the iGaming industry?
Integrating payment systems into online casinos is a complex yet vital process. By carefully analyzing requirements, selecting the right provider (considering regional differences and regulatory requirements such as AML and KYC), and implementing a robust API integration strategy, you can build a secure and efficient payment system.
Remember to leverage provider-specific libraries when available, follow best practices in code architecture (such as using design patterns for a unified interface), and maintain comprehensive logging and risk management. Optimizing queues and using test environments further ensures that your system is reliable and scalable.
By keeping up with emerging trends, including the integration of cryptocurrencies and new technologies, your casino will be well-equipped to offer a seamless, secure, and future-ready payment experience.
This article was written by Jacek Białas with substantive support of Mateusz Banaś.